Max Function In Dev C++
C: Functions We've talked a little bit about functions in the past - they're pieces of code that can be executed on command. In fact we've been using functions right since the very start of this process, we just haven't really talked about them in depth - this is what this tutorial is all about. Dec 27, 2015 In this video, an algorithm for finding the largest or smallest value in an array is shared. Then an implementation of the algorithm is done in C in a. Oct 18, 2007 Suppose we have to add two basic statistics functions using LinkList, these functions description is given below, Our first function is, Mean: Description: This function will calculate mean of the all the elements present in the list and will show the result. Its formula is, Mean = Total of all elements of the list / Length of the list. برنامج 3utools للماك. 20 rows Oct 21, 2019 C Integer Limits. The limits for integer types are listed in the following. Oh by the way, two functions are taken from the C 11: minmax and minmaxelement. The minmax is a unique function to retrieve min and max values from 2 values or from an entire array. With the keyword auto we don't need to tell what is the type of each element. Min and max of numbers read from a file. Ask Question Asked 4 years, 1 month ago. Therefore return 0 on successful program termination. Or just omit the return altogether, return 0; is implied in the main function in C. Or, if you want to be really beyond reproach. Assign min and max to avoid the need for special treatment during the.
Language | ||||
Standard Library Headers | ||||
Freestanding and hosted implementations | ||||
Named requirements | ||||
Language support library | ||||
Concepts library(C++20) | ||||
Diagnostics library | ||||
Utilities library | ||||
Strings library | ||||
Containers library | ||||
Iterators library | ||||
Ranges library(C++20) | ||||
Algorithms library | ||||
Numerics library | ||||
Input/output library | ||||
Localizations library | ||||
Regular expressions library(C++11) | ||||
Atomic operations library(C++11) | ||||
Thread support library(C++11) | ||||
Filesystem library(C++17) | ||||
Technical Specifications |
Constrained algorithms and algorithms on ranges (C++20) | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Concepts and utilities: std::sortable , std::projected , .. | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Constrained algorithms: std::ranges::copy , std::ranges::sort , .. | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Execution policies (C++17) | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Non-modifying sequence operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
|
|
| ||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Modifying sequence operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
|
|
|
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||
Operations on uninitialized storage | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
|
|
|
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||
Partitioning operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
| ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Sorting operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
| ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Binary search operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Set operations (on sorted ranges) | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Heap operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
| ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Minimum/maximum operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
| ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Permutations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
| ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Numeric operations | ||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
|
|
|
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||
C library |
C Programming Max Function
Defined in header <algorithm> | |
(1) | |
template<class T > const T& max(const T& a, const T& b ); | (until C++14) |
template<class T > constexprconst T& max(const T& a, const T& b ); | (since C++14) |
(2) | |
template<class T, class Compare > const T& max(const T& a, const T& b, Compare comp ); | (until C++14) |
template<class T, class Compare > constexprconst T& max(const T& a, const T& b, Compare comp ); | (since C++14) |
(3) | |
template<class T > T max(std::initializer_list<T> ilist ); | (since C++11) (until C++14) |
template<class T > constexpr T max(std::initializer_list<T> ilist ); | (since C++14) |
(4) | |
template<class T, class Compare > T max(std::initializer_list<T> ilist, Compare comp ); | (since C++11) (until C++14) |
template<class T, class Compare > constexpr T max(std::initializer_list<T> ilist, Compare comp ); | (since C++14) |
Returns the greater of the given values.
ilist
.The (1,3) versions use operator< to compare the values, the (2,4) versions use the given comparison function comp
.
Dev C++ Programs
[edit]Parameters
a, b | - | the values to compare |
ilist | - | initializer list with the values to compare |
comp | - | comparison function object (i.e. an object that satisfies the requirements of Compare) which returns true if a is less than b . The signature of the comparison function should be equivalent to the following: bool cmp(const Type1 &a, const Type2 &b); While the signature does not need to have const&, the function must not modify the objects passed to it and must be able to accept all values of type (possibly const) |
Type requirements | ||
-T must meet the requirements of LessThanComparable in order to use overloads (1,3). | ||
-T must meet the requirements of CopyConstructible in order to use overloads (3,4). |
[edit]Return value
a
and b
. If they are equivalent, returns a
.ilist
. If several values are equivalent to the greatest, returns the leftmost one.[edit]Complexity
ilist.size() - 1
comparisons[edit]Possible implementation
First version |
---|
Second version |
Third version |
Fourth version |
[edit]Notes
Capturing the result of std::max
by reference if one of the parameters is rvalue produces a dangling reference if that parameter is returned:
[edit]Example
Output:
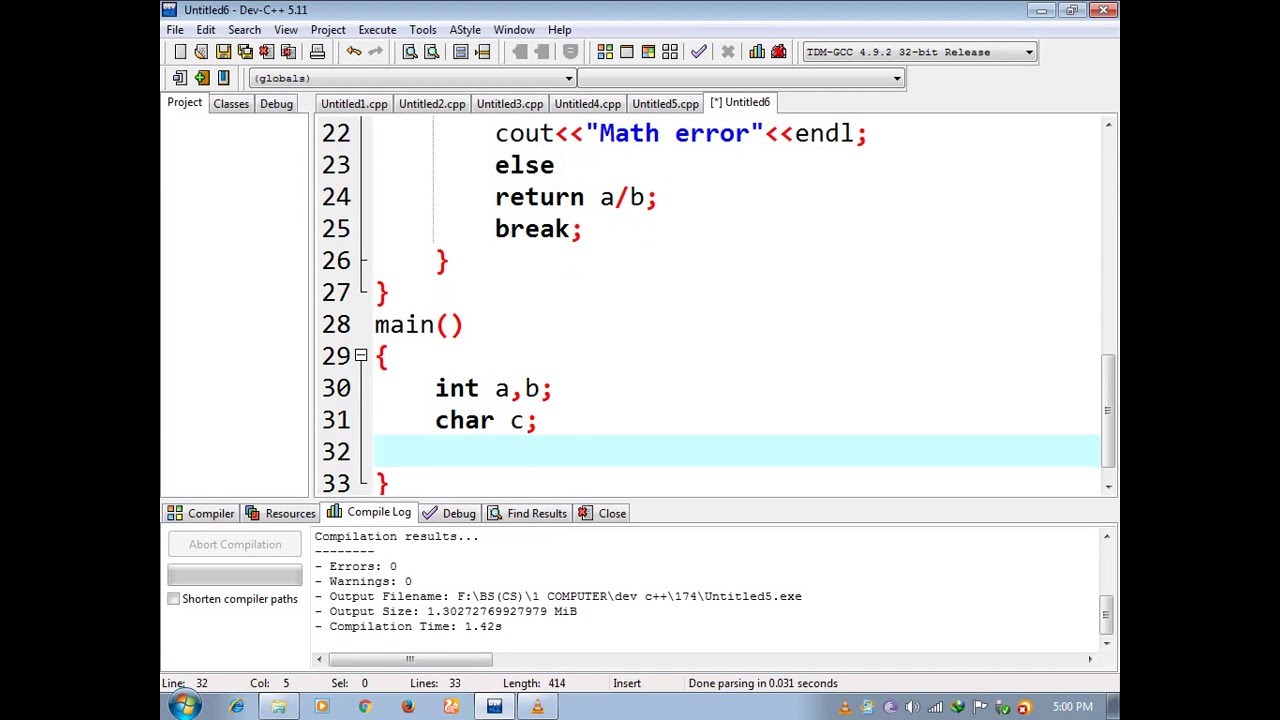
[edit]See also
returns the smaller of the given values (function template)[edit] | |
(C++11) | returns the smaller and larger of two elements (function template)[edit] |
returns the largest element in a range (function template)[edit] | |
(C++17) | clamps a value between a pair of boundary values (function template)[edit] |